I was in the search of a light-weight lightbox. I wanted it to be able to have two different images; "medium" and a link to the original. That was it, no other fancy-stuff. Finding a light-box JavaScript is super-easy. There are several choices, with or without JQuery.
But of-course, I never found one that suited my needs. Either too big or not able too handle what I wanted. So I wrote one instead. The Internet is full of custom lightbox-code, so finding inspiration was easy enough.
Olivia-box
I call it "Olivia-box". :-) It had to have a name! The code is super-easy and quite small (2kb NOT minified + couple of lines of CSS).
It basically only "dims" the screen, opens a window in the center, shows the image and a link to the original.
Also, it uses JQuery, so don't forget to include that.
Demo
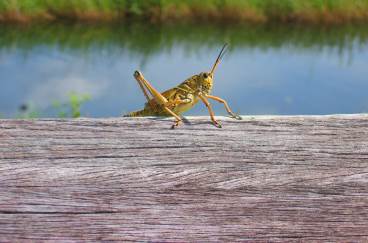
- To use it, add a link like so:
<a href="javascript:void()" data-mediumflickr="http://farm4.staticflickr.com/3799/9777395311_4499465249.jpg" data-largeflickr="http://farm4.staticflickr.com/3799/9777395311_85ddd22083_o.jpg" class="image featured"><img src="images/car01.jpg" alt="" /></a>
- The "data-mediumflickr" attribute is the link to the medium-sized image. The "data-largeflickr" attribute is the link to the original image. The other stuff is just to make the link look nicer. Result is below:
-
If we include the "oliviabox.js" it will recognize all links with the attribute "data-mediumflickr", and
put a click-event on it, calling "showOliviabox(...)". "showOliviabox" takes the attributes and builds the content. Easy, code below.
var oliviabox = (function(){
var
method = {},
$dimmer,
$obox,
$content;
method.center = function () {
var top, left;
top = Math.max($(window).height() - $obox.outerHeight(), 0) / 2;
left = Math.max($(window).width() - $obox.outerWidth(), 0) / 2;
$obox.css({
top: top + $(window).scrollTop(),
left: left + $(window).scrollLeft()
});
};
method.open = function (settings) {
$content.empty().append(settings.content);
$obox.css({
width: settings.width || 'auto',
height: settings.height || 'auto'
});
method.center();
$(window).bind('resize.obox', method.center);
$obox.show();
$dimmer.show();
};
method.close = function () {
$obox.hide();
$dimmer.hide();
$content.empty();
$(window).unbind('resize.obox');
};
$dimmer = $('<div id="dimmer"></div>');
$obox = $('<div id="obox"></div>');
$content = $('<div id="content"></div>');
$obox.hide();
$dimmer.hide();
$obox.append($content, '');
$(document).ready(function(){
$('body').append($dimmer, $obox);
});
$dimmer.click(function(e){
e.preventDefault();
method.close();
});
return method;
}());
// Init
function showOliviabox(el) {
var srcToMedImg = el.getAttribute('data-mediumflickr');
var linkToLargeImg = el.getAttribute('data-largeflickr');
oliviabox.open({content: "<img src='"+srcToMedImg+
"' /><div><a href='"+linkToLargeImg+"' target='_blank'>Open large</a>
</div>",width: "550px", height: "480px"});
return false;
}
$(document).ready(function(){
$( "[data-mediumflickr]" ).on( "click", function() {
showOliviabox(this);
});
}); -
The CSS used for this:
/********************************************************/ /* Olivia Box /********************************************************/ #dimmer { position:fixed; top:0; left:0; width:100%; height:100%; background:#000; opacity:0.5; filter:alpha(opacity=50); } #obox { position:absolute; background:rgba(0,0,0,0.2); border-radius:14px; padding:8px; } #content { border-radius:8px; background:#fff; padding:20px; }